Tooltip
IObservable
or INotifyPropertyChanged
interface.Using Tooltip
Add tooltip to gameobject:
Tooltip.Register(
TargetGO, // gameobject to add tooltip
Data, // data to display
new TooltipSettings(TooltipPosition.TopCenter, delay = 0.3f, unscaledTime = true)
);
Remove tooltip:
Tooltip.Unregister(TargetGO);
Also tooltip can be added with Tooltip Viewer component.
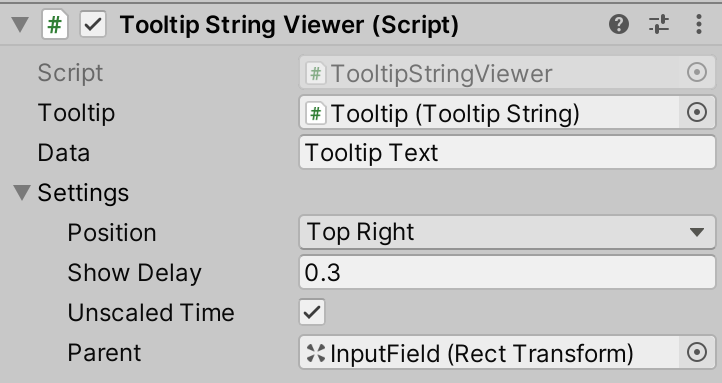
Destroy Tooltip Viewer component to remove tooltip.
Tooltip Fields and Properties
TData
CurrentDataGameObject
CurrentTarget
Tooltip Methods
Register(
GameObject
target,TData
data,TooltipSettings
settings)Unregister(
GameObject
target)Show(
GameObject
target)Hide()
TData
GetData(GameObject
target)bool
UpdateData(GameObject
target,TData
data)TooltipSettings
GetSettings(GameObject
target)UpdateSettings(
GameObject
target,TooltipSettings
settings)
Tooltip Events
OnShow
UnityEvent<TTooltip, GameObject>
OnHide
UnityEvent<TTooltip, GameObject>
Tooltip Settings
Position
TooltipPosition
Tooltip position relative to target gameobject.
Delay
float
Delay before tooltip displayed.
Parent
RectTransform
Tooltip parent.
UnscaledTime
bool
Delay specified in unscaled time.
TooltipPosition
Top Left
Top Center
Top Right
Middle Left
Middle Center
Middle Right
Bottom Left
Bottom Center
Bottom Right
Tooltip Viewer Fields
Tooltip
TTooltip
Data
TData
Data to display.
Settings
TooltipSettings
Tooltip display settings.
Tooltip Code Example
namespace UIWidgets
{
/// <summary>
/// Tooltip string.
/// </summary>
public class TooltipString : Tooltip<string, TooltipString>
{
/// <summary>
/// Text.
/// </summary>
public TextAdapter Text;
/// <summary>
/// Item.
/// </summary>
public string Item
{
get;
protected set;
}
/// <inheritdoc/>
protected override void SetData(string data)
{
Item = data;
UpdateView();
}
/// <inheritdoc/>
protected override void UpdateView()
{
Text.text = Item;
}
}
}
Tooltip Viewer Code Example
namespace UIWidgets
{
/// <summary>
/// TooltipString viewer.
/// </summary>
public class TooltipStringViewer : TooltipViewer<string, TooltipString>
{
}
}