Custom Widgets
By default, the properties of components are controlled by Theme Target, which is not always desirable when using the Attach Theme context menu, for example, if the image color is controlled by a widget and you don’t want to manually disable it for each such component.
To avoid this, you can use the
UIThemes.Utilities.SetTargetOwner<TComponent>(Type propertyType, TComponent component, string property, Component owner)
method to indicate that the properties of the specified component are controlled by widget.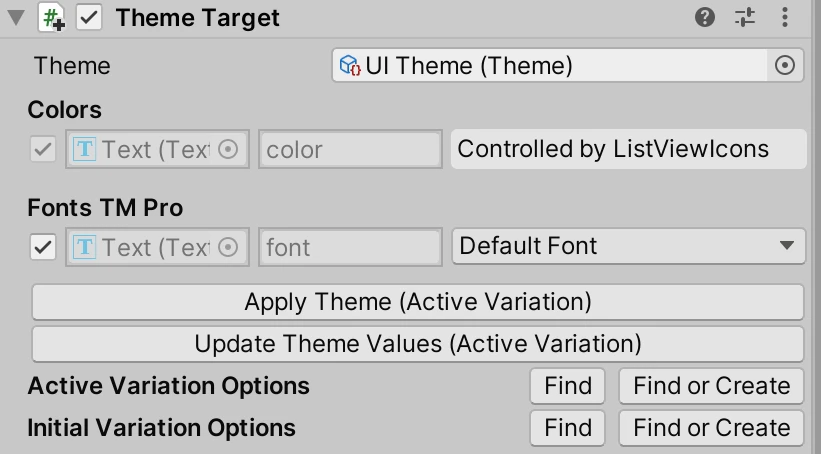
The font color property is controlled by ListViewIcons and cannot be changed. On click, ListViewIcons will be highlighted in the Hierarchy window.
Original Widget Code
using UIThemes;
using UnityEngine;
using UnityEngine.UI;
// this widget changes image color when the toggle value is changed
public class ToggleBackgroundController : MonoBehaviour
{
public Toggle Toggle;
public Image ToggleBackground;
[SerializeField]
Color colorOn = Color.white;
public Color ColorOn
{
get => colorOn;
set
{
colorOn = value;
UpdateColor(Toggle.isOn);
}
}
[SerializeField]
Color colorOff = Color.white;
public Color ColorOff
{
get => colorOff;
set
{
colorOff = value;
UpdateColor(Toggle.isOn);
}
}
void Start()
{
Toggle.onValueChanged.AddListener(UpdateColor);
UpdateColor(Toggle.isOn);
}
void OnDestroy() => Toggle.onValueChanged.RemoveListener(UpdateColor);
void UpdateColor(bool isOn) => ToggleBackground.color = isOn ? colorOn : colorOff;
}
Widget Code Changes
// added methods SetImageOwner() and OnValidate()
void SetImageOwner() => UIThemes.Utilities.SetTargetOwner<Graphic>(typeof(Color), ToggleBackground, nameof(Image.color), this);
#if UNITY_EDITOR
void OnValidate() => SetImageOwner();
#endif
void Start()
{
SetImageOwner(); // added line
Toggle.onValueChanged.AddListener(UpdateColor);
UpdateColor(Toggle.isOn);
}